Input And Output Devices
PPM SENSOR
For this i have used PMS 7003 high effeciency ppm sensor
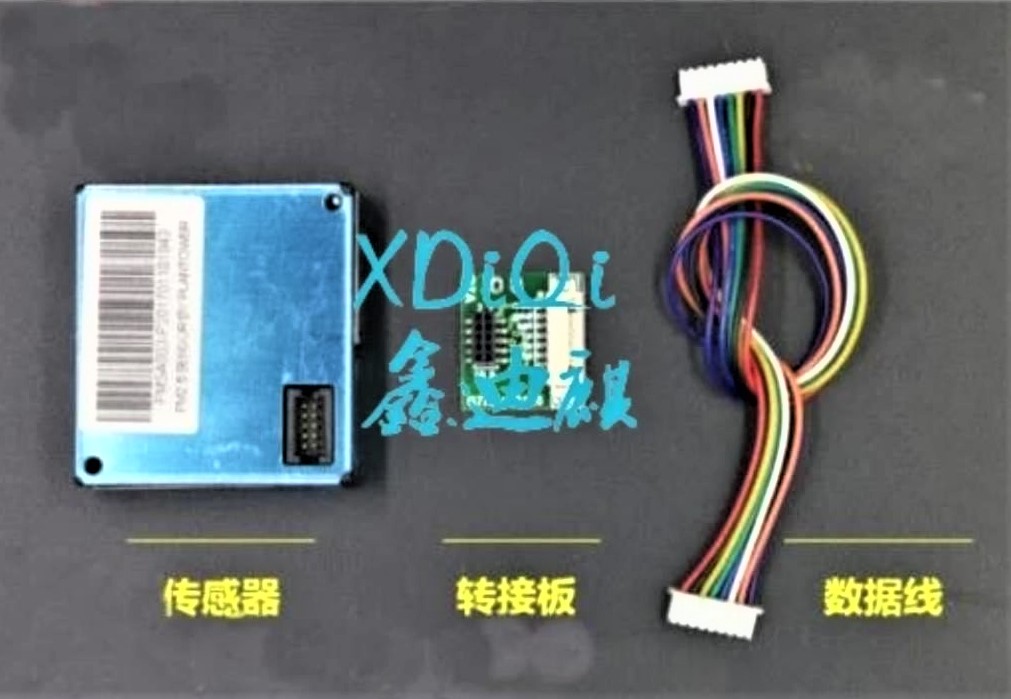
If you woul like to know how i integerated that pls click on this link, this link will guide you to my input devices page there i have in detail explained how you can integerate the sensor , as well as i have provided the appropriate programming as well all the links for tutorial and sites from where you can learn more about this sensor
Purpose of my listing this thing here is to give an overview of things before i can add more complex data
PPM SENSOR with fan
In previouse weeks i have listed the method of controlling the speed of Brushless D.C fan using PWM If you want to know more about that please click on this link,
Now i am going to list the code for PPM sensor controlled D.C Brushless
#include "SoftwareSerial.h" SoftwareSerial pmsSerial(2, 3); #define motorPin 11 // variable declaration // const value will not change const int fan=11; // fan connected to PWM11 on Arduino pin 11 int pms_interval_time=5000; // PMS5003 delay time between taking next reading, 1000 = 1sec void setup() { // our debugging output Serial.begin(115200); // sensor baud rate is 9600 pmsSerial.begin(9600); //define motor pin as an output pinMode(motorPin, OUTPUT); } struct pms7003data { uint16_t framelen; uint16_t pm10_standard, pm25_standard, pm100_standard; uint16_t pm10_env, pm25_env, pm100_env; uint16_t particles_03um, particles_05um, particles_10um, particles_25um, particles_50um, particles_100um; uint16_t unused; uint16_t checksum; }; struct pms7003data data; void loop() { if (readPMSdata(&pmsSerial)) { //reading data was successful! Serial.println(); Serial.println("---------------------------------------"); Serial.println("Concentration Units (standard)"); Serial.print("PM 1.0: "); Serial.print(data.pm10_standard); Serial.print("\t\tPM 2.5: "); Serial.print(data.pm25_standard); Serial.print("\t\tPM 10: "); Serial.println(data.pm100_standard); Serial.println("---------------------------------------"); // Sound off buzzer if PM2.5 or PM10 read the 'moderate polluted range' // Good : PM10 (1-50) PM2.5(0-30) // Satisfactory : PM10 (51-100) PM2.5(31-60) // Moderately polluted : PM10 (101-250) PM2.5(61-90) // Poor : PM10 (251-350) PM2.5(91-120) // Very poor : PM10 (351-430) PM2.5(121-250) // Severe : PM10 (430+) PM2.5(250+) if (data.pm100_standard >= 151) { analogWrite(fan,HIGH); } else { analogWrite(fan,LOW); } if (data.pm25_standard >= 91) { digitalWrite(fan,HIGH); } else { digitalWrite(fan,LOW); } } } boolean readPMSdata(Stream *s) { if (! s->available()) { return false; } // Read a byte at a time until we get to the special '0x42' start-byte if (s->peek() != 0x42) { s->read(); return false; } // Now read all 32 bytes if (s->available() < 32) { return false; } uint8_t buffer[32]; uint16_t sum = 0; s->readBytes(buffer, 32); // get checksum ready for (uint8_t i=0; i<30; i++) { sum += buffer[i]; } /* debugging for (uint8_t i=2; i<32; i++) { Serial.print("0x"); Serial.print(buffer[i], HEX); Serial.print(", "); } Serial.println(); */ // The data comes in endian'd, this solves it so it works on all platforms uint16_t buffer_u16[15]; for (uint8_t i=0; i<15; i++) { buffer_u16[i] = buffer[2 + i*2 + 1]; buffer_u16[i] += (buffer[2 + i*2] << 8); } // put it into a nice struct :) memcpy((void *)&data, (void *)buffer_u16, 30); if (sum != data.checksum) { Serial.println("Checksum failure"); return false; } // success! return true; }
And if you want to download the CODE ,you can click on this link for that
PPM_sensor_with_fanThe above code is very simple i have taken both the codes from input and output device week and merge them in the sink
Let me explain what the code suppose to do , i have written that if the PPM level increases a certain lvl then speed of fan is suppose to increase with it
i.e. What you can say , make this air filter a smart air filter as this smartly control the power consumption according to its requirement
2.4 inch TFT screen
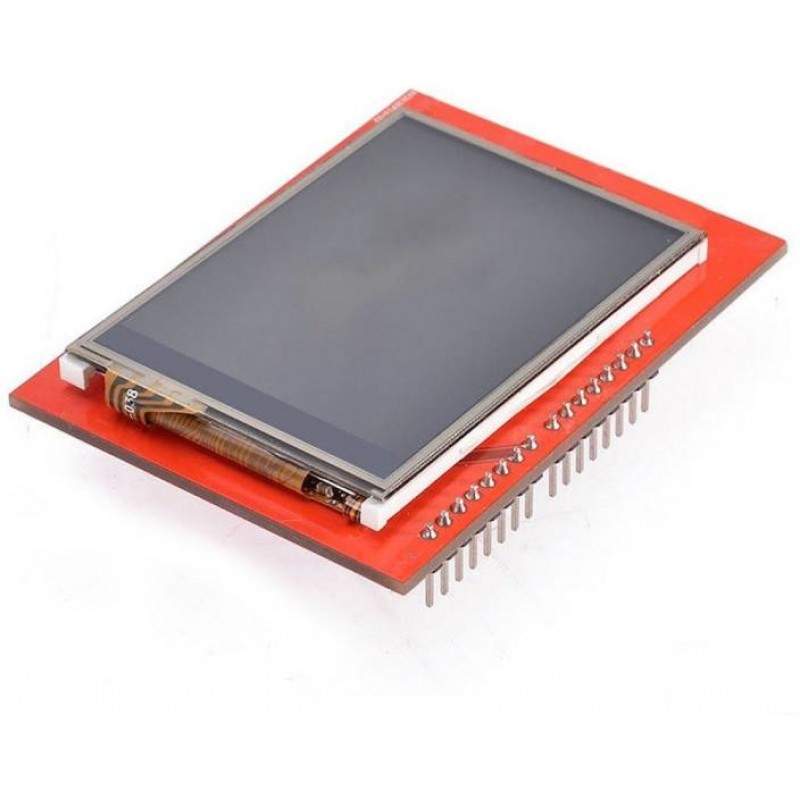
This is the image of an2.4 inch tft screen which i bought locally
My purpose of adding this screen here was ,as i have explained that i have planned to isplay all the sensor data on the screen and for this purpose i have choosed this screen
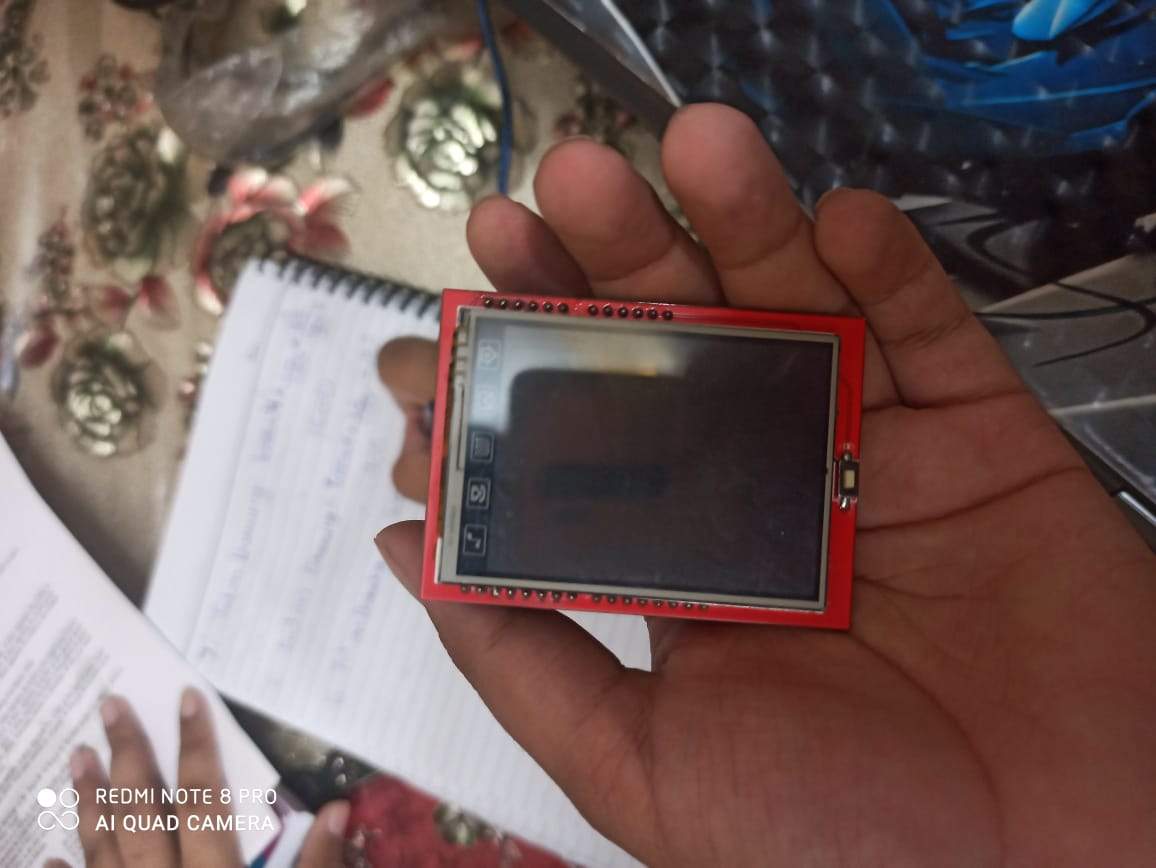
It is half of size of my hand as you can see
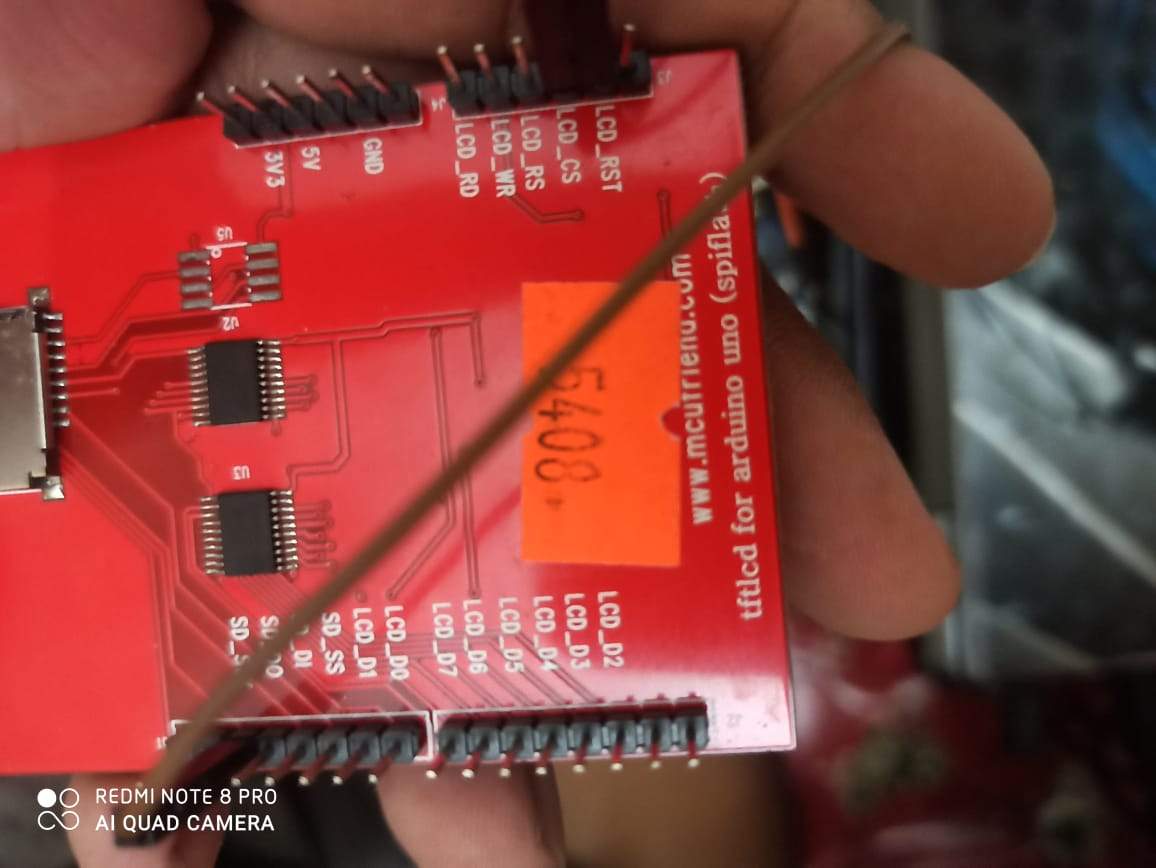
From This image you can see it has number pins on it
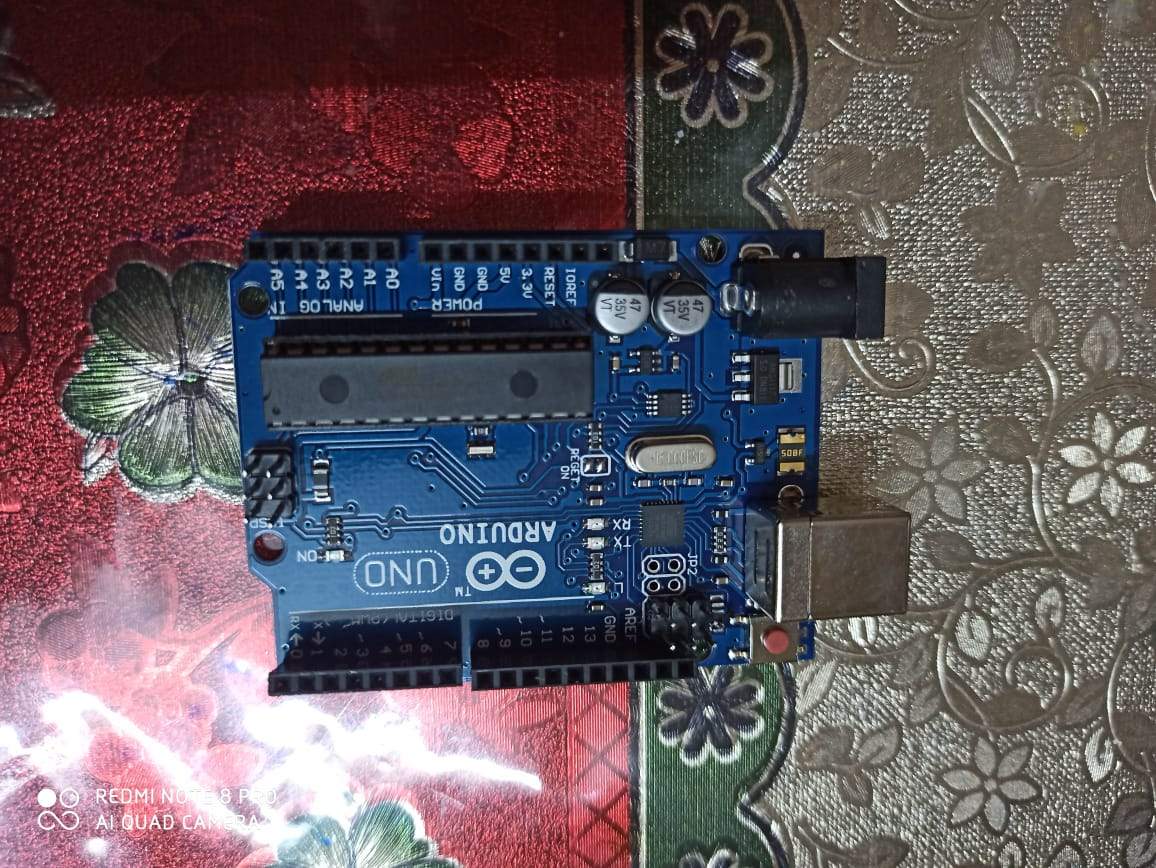
Well this screen is made exactly to fit on the arduino just like a shield
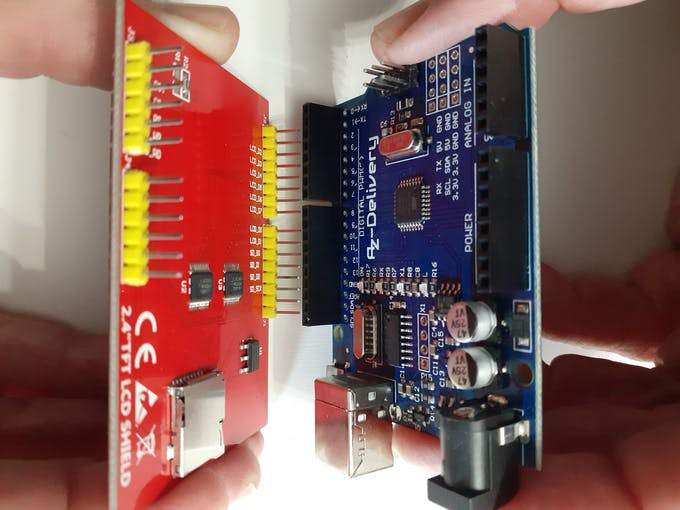
Well this screen is made exactly to fit on the arduino just like a shield
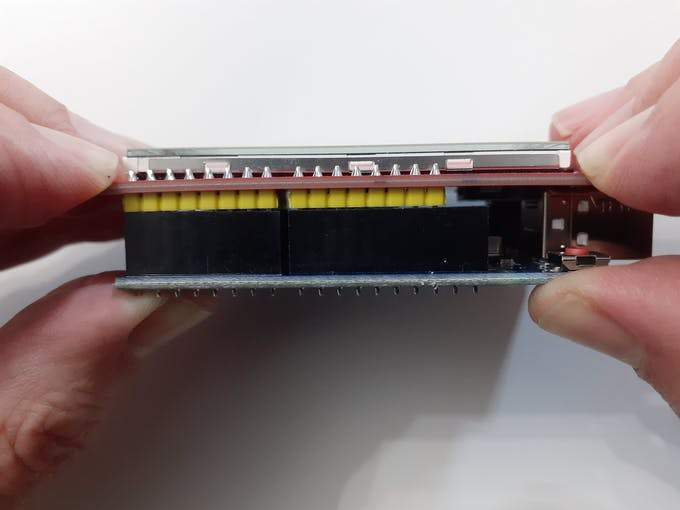
Please place the screen on the top of the sheild properly by matching the pin correctly , otherwise you can cause damage to the sheild
I am providing link to the perfect tutorial from where i have learned how to use the tft 2.4 inch screen as there were very less tutorial online for this device , due to its driver , as i also struggle to find the appropriate tutorial to use it
Testing the screen
// All the mcufriend.com UNO shields have the same pinout. // i.e. control pins A0-A4. Data D2-D9. microSD D10-D13. // Touchscreens are normally A1, A2, D7, D6 but the order varies // // This demo should work with most Adafruit TFT libraries // If you are not using a shield, use a full Adafruit constructor() // e.g. Adafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET); #define LCD_CS A3 // Chip Select goes to Analog 3 #define LCD_CD A2 // Command/Data goes to Analog 2 #define LCD_WR A1 // LCD Write goes to Analog 1 #define LCD_RD A0 // LCD Read goes to Analog 0 #define LCD_RESET A4 // Can alternately just connect to Arduino's reset pin #include// f.k. for Arduino-1.5.2 #include "Adafruit_GFX.h"// Hardware-specific library #include MCUFRIEND_kbv tft; //#include //Adafruit_TFTLCD tft(LCD_CS, LCD_CD, LCD_WR, LCD_RD, LCD_RESET); // Assign human-readable names to some common 16-bit color values: #define BLACK 0x0000 #define BLUE 0x001F #define RED 0xF800 #define GREEN 0x07E0 #define CYAN 0x07FF #define MAGENTA 0xF81F #define YELLOW 0xFFE0 #define WHITE 0xFFFF #ifndef min #define min(a, b) (((a) < (b)) ? (a) : (b)) #endif void setup(void); void loop(void); unsigned long testFillScreen(); unsigned long testText(); unsigned long testLines(uint16_t color); unsigned long testFastLines(uint16_t color1, uint16_t color2); unsigned long testRects(uint16_t color); unsigned long testFilledRects(uint16_t color1, uint16_t color2); unsigned long testFilledCircles(uint8_t radius, uint16_t color); unsigned long testCircles(uint8_t radius, uint16_t color); unsigned long testTriangles(); unsigned long testFilledTriangles(); unsigned long testRoundRects(); unsigned long testFilledRoundRects(); void progmemPrint(const char *str); void progmemPrintln(const char *str); void runtests(void); uint16_t g_identifier; extern const uint8_t hanzi[]; void showhanzi(unsigned int x, unsigned int y, unsigned char index) { uint8_t i, j, c, first = 1; uint8_t *temp = (uint8_t*)hanzi; uint16_t color; tft.setAddrWindow(x, y, x + 31, y + 31); //设置区域 temp += index * 128; for (j = 0; j < 128; j++) { c = pgm_read_byte(temp); for (i = 0; i < 8; i++) { if ((c & (1 << i)) != 0) { color = RED; } else { color = BLACK; } tft.pushColors(&color, 1, first); first = 0; } temp++; } } void setup(void) { Serial.begin(9600); uint32_t when = millis(); // while (!Serial) ; //hangs a Leonardo until you connect a Serial if (!Serial) delay(5000); //allow some time for Leonardo Serial.println("Serial took " + String((millis() - when)) + "ms to start"); // tft.reset(); //hardware reset uint16_t ID = tft.readID(); // Serial.print("ID = 0x"); Serial.println(ID, HEX); if (ID == 0xD3D3) ID = 0x9481; // write-only shield // ID = 0x9329; // force ID tft.begin(ID); } #if defined(MCUFRIEND_KBV_H_) uint16_t scrollbuf[320]; // my biggest screen is 320x480 #define READGRAM(x, y, buf, w, h) tft.readGRAM(x, y, buf, w, h) #else uint16_t scrollbuf[320]; // Adafruit only does 240x320 // Adafruit can read a block by one pixel at a time int16_t READGRAM(int16_t x, int16_t y, uint16_t *block, int16_t w, int16_t h) { uint16_t *p; for (int row = 0; row < h; row++) { p = block + row * w; for (int col = 0; col < w; col++) { *p++ = tft.readPixel(x + col, y + row); } } } #endif void windowScroll(int16_t x, int16_t y, int16_t wid, int16_t ht, int16_t dx, int16_t dy, uint16_t *buf) { if (dx) for (int16_t row = 0; row < ht; row++) { READGRAM(x, y + row, buf, wid, 1); tft.setAddrWindow(x, y + row, x + wid - 1, y + row); tft.pushColors(buf + dx, wid - dx, 1); tft.pushColors(buf + 0, dx, 0); } if (dy) for (int16_t col = 0; col < wid; col++) { READGRAM(x + col, y, buf, 1, ht); tft.setAddrWindow(x + col, y, x + col, y + ht - 1); tft.pushColors(buf + dy, ht - dy, 1); tft.pushColors(buf + 0, dy, 0); } } void printmsg(int row, const char *msg) { tft.setTextColor(YELLOW, BLACK); tft.setCursor(0, row); tft.println(msg); } void loop(void) { uint8_t aspect; uint16_t pixel; const char *aspectname[] = { "PORTRAIT", "LANDSCAPE", "PORTRAIT_REV", "LANDSCAPE_REV" }; const char *colorname[] = { "BLUE", "GREEN", "RED", "GRAY" }; uint16_t colormask[] = { 0x001F, 0x07E0, 0xF800, 0xFFFF }; uint16_t dx, rgb, n, wid, ht, msglin; tft.setRotation(0); runtests(); delay(2000); if (tft.height() > 64) { for (uint8_t cnt = 0; cnt < 4; cnt++) { aspect = (cnt + 0) & 3; tft.setRotation(aspect); wid = tft.width(); ht = tft.height(); msglin = (ht > 160) ? 200 : 112; testText(); dx = wid / 32; for (n = 0; n < 32; n++) { rgb = n * 8; rgb = tft.color565(rgb, rgb, rgb); tft.fillRect(n * dx, 48, dx, 63, rgb & colormask[aspect]); } tft.drawRect(0, 48 + 63, wid, 1, WHITE); tft.setTextSize(2); tft.setTextColor(colormask[aspect], BLACK); tft.setCursor(0, 72); tft.print(colorname[aspect]); tft.setTextColor(WHITE); tft.println(" COLOR GRADES"); tft.setTextColor(WHITE, BLACK); printmsg(184, aspectname[aspect]); delay(1000); tft.drawPixel(0, 0, YELLOW); pixel = tft.readPixel(0, 0); tft.setTextSize((ht > 160) ? 2 : 1); //for messages #if defined(MCUFRIEND_KBV_H_) #if 1 extern const uint8_t penguin[]; tft.setAddrWindow(wid - 40 - 40, 20 + 0, wid - 1 - 40, 20 + 39); tft.pushColors(penguin, 1600, 1); #elif 1 extern const uint8_t wifi_full[]; tft.setAddrWindow(wid - 40 - 40, 20 + 0, wid - 40 - 40 + 31, 20 + 31); tft.pushColors(wifi_full, 1024, 1, true); #elif 1 extern const uint8_t icon_40x40[]; tft.setAddrWindow(wid - 40 - 40, 20 + 0, wid - 1 - 40, 20 + 39); tft.pushColors(icon_40x40, 1600, 1); #endif tft.setAddrWindow(0, 0, wid - 1, ht - 1); if (aspect & 1) tft.drawRect(wid - 1, 0, 1, ht, WHITE); else tft.drawRect(0, ht - 1, wid, 1, WHITE); printmsg(msglin, "VERTICAL SCROLL UP"); uint16_t maxscroll; if (tft.getRotation() & 1) maxscroll = wid; else maxscroll = ht; for (uint16_t i = 1; i <= maxscroll; i++) { tft.vertScroll(0, maxscroll, i); delay(10); } delay(1000); printmsg(msglin, "VERTICAL SCROLL DN"); for (uint16_t i = 1; i <= maxscroll; i++) { tft.vertScroll(0, maxscroll, 0 - (int16_t)i); delay(10); } tft.vertScroll(0, maxscroll, 0); printmsg(msglin, "SCROLL DISABLED "); delay(1000); if ((aspect & 1) == 0) { //Portrait tft.setTextColor(BLUE, BLACK); printmsg(msglin, "ONLY THE COLOR BAND"); for (uint16_t i = 1; i <= 64; i++) { tft.vertScroll(48, 64, i); delay(20); } delay(1000); } #endif tft.setTextColor(YELLOW, BLACK); if (pixel == YELLOW) { printmsg(msglin, "SOFTWARE SCROLL "); #if 0 // diagonal scroll of block for (int16_t i = 45, dx = 2, dy = 1; i > 0; i -= dx) { windowScroll(24, 8, 90, 40, dx, dy, scrollbuf); } #else // plain horizontal scroll of block n = (wid > 320) ? 320 : wid; for (int16_t i = n, dx = 4, dy = 0; i > 0; i -= dx) { windowScroll(0, 200, n, 16, dx, dy, scrollbuf); } #endif } else if (pixel == CYAN) tft.println("readPixel() reads as BGR"); else if ((pixel & 0xF8F8) == 0xF8F8) tft.println("readPixel() should be 24-bit"); else { tft.print("readPixel() reads 0x"); tft.println(pixel, HEX); } delay(5000); } } printmsg(msglin, "INVERT DISPLAY "); tft.invertDisplay(true); delay(2000); tft.invertDisplay(false); } typedef struct { PGM_P msg; uint32_t ms; } TEST; TEST result[12]; #define RUNTEST(n, str, test) { result[n].msg = PSTR(str); result[n].ms = test; delay(500); } void runtests(void) { uint8_t i, len = 24, cnt; uint32_t total; RUNTEST(0, "FillScreen ", testFillScreen()); RUNTEST(1, "Text ", testText()); RUNTEST(2, "Lines ", testLines(CYAN)); RUNTEST(3, "Horiz/Vert Lines ", testFastLines(RED, BLUE)); RUNTEST(4, "Rectangles (outline) ", testRects(GREEN)); RUNTEST(5, "Rectangles (filled) ", testFilledRects(YELLOW, MAGENTA)); RUNTEST(6, "Circles (filled) ", testFilledCircles(10, MAGENTA)); RUNTEST(7, "Circles (outline) ", testCircles(10, WHITE)); RUNTEST(8, "Triangles (outline) ", testTriangles()); RUNTEST(9, "Triangles (filled) ", testFilledTriangles()); RUNTEST(10, "Rounded rects (outline) ", testRoundRects()); RUNTEST(11, "Rounded rects (filled) ", testFilledRoundRects()); tft.fillScreen(BLACK); tft.setTextColor(GREEN); tft.setCursor(0, 0); uint16_t wid = tft.width(); if (wid > 176) { tft.setTextSize(2); #if defined(MCUFRIEND_KBV_H_) tft.print("MCUFRIEND "); #if MCUFRIEND_KBV_H_ != 0 tft.print(0.01 * MCUFRIEND_KBV_H_, 2); #else tft.print("for"); #endif tft.println(" UNO"); #else tft.println("Adafruit-Style Tests"); #endif } else len = wid / 6 - 8; tft.setTextSize(1); total = 0; for (i = 0; i < 12; i++) { PGM_P str = result[i].msg; char c; if (len > 24) { if (i < 10) tft.print(" "); tft.print(i); tft.print(": "); } uint8_t cnt = len; while ((c = pgm_read_byte(str++)) && cnt--) tft.print(c); tft.print(" "); tft.println(result[i].ms); total += result[i].ms; } tft.setTextSize(2); tft.print("Total:"); tft.print(0.000001 * total); tft.println("sec"); g_identifier = tft.readID(); tft.print("ID: 0x"); tft.println(tft.readID(), HEX); // tft.print("Reg(00):0x"); // tft.println(tft.readReg(0x00), HEX); tft.print("F_CPU:"); tft.print(0.000001 * F_CPU); #if defined(__OPTIMIZE_SIZE__) tft.println("MHz -Os"); #else tft.println("MHz"); #endif delay(10000); } // Standard Adafruit tests. will adjust to screen size unsigned long testFillScreen() { unsigned long start = micros(); tft.fillScreen(BLACK); tft.fillScreen(RED); tft.fillScreen(GREEN); tft.fillScreen(BLUE); tft.fillScreen(BLACK); return micros() - start; } unsigned long testText() { unsigned long start; tft.fillScreen(BLACK); start = micros(); tft.setCursor(0, 0); tft.setTextColor(WHITE); tft.setTextSize(1); tft.println("Hello World!"); tft.setTextColor(YELLOW); tft.setTextSize(2); tft.println(123.45); tft.setTextColor(RED); tft.setTextSize(3); tft.println(0xDEADBEEF, HEX); tft.println(); tft.setTextColor(GREEN); tft.setTextSize(5); tft.println("Groop"); tft.setTextSize(2); tft.println("I implore thee,"); tft.setTextSize(1); tft.println("my foonting turlingdromes."); tft.println("And hooptiously drangle me"); tft.println("with crinkly bindlewurdles,"); tft.println("Or I will rend thee"); tft.println("in the gobberwarts"); tft.println("with my blurglecruncheon,"); tft.println("see if I don't!"); return micros() - start; } unsigned long testLines(uint16_t color) { unsigned long start, t; int x1, y1, x2, y2, w = tft.width(), h = tft.height(); tft.fillScreen(BLACK); x1 = y1 = 0; y2 = h - 1; start = micros(); for (x2 = 0; x2 < w; x2 += 6) tft.drawLine(x1, y1, x2, y2, color); x2 = w - 1; for (y2 = 0; y2 < h; y2 += 6) tft.drawLine(x1, y1, x2, y2, color); t = micros() - start; // fillScreen doesn't count against timing tft.fillScreen(BLACK); x1 = w - 1; y1 = 0; y2 = h - 1; start = micros(); for (x2 = 0; x2 < w; x2 += 6) tft.drawLine(x1, y1, x2, y2, color); x2 = 0; for (y2 = 0; y2 < h; y2 += 6) tft.drawLine(x1, y1, x2, y2, color); t += micros() - start; tft.fillScreen(BLACK); x1 = 0; y1 = h - 1; y2 = 0; start = micros(); for (x2 = 0; x2 < w; x2 += 6) tft.drawLine(x1, y1, x2, y2, color); x2 = w - 1; for (y2 = 0; y2 < h; y2 += 6) tft.drawLine(x1, y1, x2, y2, color); t += micros() - start; tft.fillScreen(BLACK); x1 = w - 1; y1 = h - 1; y2 = 0; start = micros(); for (x2 = 0; x2 < w; x2 += 6) tft.drawLine(x1, y1, x2, y2, color); x2 = 0; for (y2 = 0; y2 < h; y2 += 6) tft.drawLine(x1, y1, x2, y2, color); return micros() - start; } unsigned long testFastLines(uint16_t color1, uint16_t color2) { unsigned long start; int x, y, w = tft.width(), h = tft.height(); tft.fillScreen(BLACK); start = micros(); for (y = 0; y < h; y += 5) tft.drawFastHLine(0, y, w, color1); for (x = 0; x < w; x += 5) tft.drawFastVLine(x, 0, h, color2); return micros() - start; } unsigned long testRects(uint16_t color) { unsigned long start; int n, i, i2, cx = tft.width() / 2, cy = tft.height() / 2; tft.fillScreen(BLACK); n = min(tft.width(), tft.height()); start = micros(); for (i = 2; i < n; i += 6) { i2 = i / 2; tft.drawRect(cx - i2, cy - i2, i, i, color); } return micros() - start; } unsigned long testFilledRects(uint16_t color1, uint16_t color2) { unsigned long start, t = 0; int n, i, i2, cx = tft.width() / 2 - 1, cy = tft.height() / 2 - 1; tft.fillScreen(BLACK); n = min(tft.width(), tft.height()); for (i = n; i > 0; i -= 6) { i2 = i / 2; start = micros(); tft.fillRect(cx - i2, cy - i2, i, i, color1); t += micros() - start; // Outlines are not included in timing results tft.drawRect(cx - i2, cy - i2, i, i, color2); } return t; } unsigned long testFilledCircles(uint8_t radius, uint16_t color) { unsigned long start; int x, y, w = tft.width(), h = tft.height(), r2 = radius * 2; tft.fillScreen(BLACK); start = micros(); for (x = radius; x < w; x += r2) { for (y = radius; y < h; y += r2) { tft.fillCircle(x, y, radius, color); } } return micros() - start; } unsigned long testCircles(uint8_t radius, uint16_t color) { unsigned long start; int x, y, r2 = radius * 2, w = tft.width() + radius, h = tft.height() + radius; // Screen is not cleared for this one -- this is // intentional and does not affect the reported time. start = micros(); for (x = 0; x < w; x += r2) { for (y = 0; y < h; y += r2) { tft.drawCircle(x, y, radius, color); } } return micros() - start; } unsigned long testTriangles() { unsigned long start; int n, i, cx = tft.width() / 2 - 1, cy = tft.height() / 2 - 1; tft.fillScreen(BLACK); n = min(cx, cy); start = micros(); for (i = 0; i < n; i += 5) { tft.drawTriangle( cx , cy - i, // peak cx - i, cy + i, // bottom left cx + i, cy + i, // bottom right tft.color565(0, 0, i)); } return micros() - start; } unsigned long testFilledTriangles() { unsigned long start, t = 0; int i, cx = tft.width() / 2 - 1, cy = tft.height() / 2 - 1; tft.fillScreen(BLACK); start = micros(); for (i = min(cx, cy); i > 10; i -= 5) { start = micros(); tft.fillTriangle(cx, cy - i, cx - i, cy + i, cx + i, cy + i, tft.color565(0, i, i)); t += micros() - start; tft.drawTriangle(cx, cy - i, cx - i, cy + i, cx + i, cy + i, tft.color565(i, i, 0)); } return t; } unsigned long testRoundRects() { unsigned long start; int w, i, i2, red, step, cx = tft.width() / 2 - 1, cy = tft.height() / 2 - 1; tft.fillScreen(BLACK); w = min(tft.width(), tft.height()); start = micros(); red = 0; step = (256 * 6) / w; for (i = 0; i < w; i += 6) { i2 = i / 2; red += step; tft.drawRoundRect(cx - i2, cy - i2, i, i, i / 8, tft.color565(red, 0, 0)); } return micros() - start; } unsigned long testFilledRoundRects() { unsigned long start; int i, i2, green, step, cx = tft.width() / 2 - 1, cy = tft.height() / 2 - 1; tft.fillScreen(BLACK); start = micros(); green = 256; step = (256 * 6) / min(tft.width(), tft.height()); for (i = min(tft.width(), tft.height()); i > 20; i -= 6) { i2 = i / 2; green -= step; tft.fillRoundRect(cx - i2, cy - i2, i, i, i / 8, tft.color565(0, green, 0)); } return micros() - start; }
Dont get scared from this very very long code , this is the standard Graphical test which is given in the exaples of the MCUFRIEND_kbv library
My purpose of listing this code was to tell you how great great example is this code , if watch clearly how this code by deleting and adding lines you can easliy understand how to make GUI for the TFT screen
Code writing
#include "Adafruit_GFX.h" #include "MCUFRIEND_kbv.h" MCUFRIEND_kbv tft; #include "Fonts/FreeSans9pt7b.h" #include "Fonts/FreeSans12pt7b.h" #include "Fonts/FreeSerif12pt7b.h" #include "FreeDefaultFonts.h" #define PI 3.1415926535897932384626433832795 int col[8]; void showmsgXY(int x, int y, int sz, const GFXfont *f, const char *msg) { int16_t x1, y1; uint16_t wid, ht; tft.setFont(f); tft.setCursor(x, y); tft.setTextColor(0x0000); tft.setTextSize(sz); tft.print(msg); } void setup() { tft.reset(); Serial.begin(9600); uint16_t ID = tft.readID(); tft.begin(ID); tft.setRotation(1); tft.invertDisplay(true); tft.fillScreen(0xffff); showmsgXY(170, 250, 2, &FreeSans9pt7b, "Loading..."); col[0] = tft.color565(155, 0, 50); col[1] = tft.color565(170, 30, 80); col[2] = tft.color565(195, 60, 110); col[3] = tft.color565(215, 90, 140); col[4] = tft.color565(230, 120, 170); col[5] = tft.color565(250, 150, 200); col[6] = tft.color565(255, 180, 220); col[7] = tft.color565(255, 210, 240); } void loop() { for (int i = 8; i > 0; i--) { tft.fillCircle(240 + 40 * (cos(-i * PI / 4)), 120 + 40 * (sin(-i * PI / 4)), 10, col[0]); delay(15); tft.fillCircle(240 + 40 * (cos(-(i + 1)*PI / 4)), 120 + 40 * (sin(-(i + 1)*PI / 4)), 10, col[1]); delay(15); tft.fillCircle(240 + 40 * (cos(-(i + 2)*PI / 4)), 120 + 40 * (sin(-(i + 2)*PI / 4)), 10, col[2]); delay(15); tft.fillCircle(240 + 40 * (cos(-(i + 3)*PI / 4)), 120 + 40 * (sin(-(i + 3)*PI / 4)), 10, col[3]); delay(15); tft.fillCircle(240 + 40 * (cos(-(i + 4)*PI / 4)), 120 + 40 * (sin(-(i + 4)*PI / 4)), 10, col[4]); delay(15); tft.fillCircle(240 + 40 * (cos(-(i + 5)*PI / 4)), 120 + 40 * (sin(-(i + 5)*PI / 4)), 10, col[5]); delay(15); tft.fillCircle(240 + 40 * (cos(-(i + 6)*PI / 4)), 120 + 40 * (sin(-(i + 6)*PI / 4)), 10, col[6]); delay(15); tft.fillCircle(240 + 40 * (cos(-(i + 7)*PI / 4)), 120 + 40 * (sin(-(i + 7)*PI / 4)), 10, col[7]); delay(15); } }
And if you want to download the CODE ,you can click on this link for that
TFT_loading_codeThe above code is used to display the loading part which takes place at start
Final code for the screen
#include#include "Adafruit_GFX.h" // Core graphics library #include "MCUFRIEND_kbv.h" MCUFRIEND_kbv tft; #define LCD_CS A3 // Chip Select goes to Analog 3 #define LCD_CD A2 // Command/Data goes to Analog 2 #define LCD_WR A1 // LCD Write goes to Analog 1 #define LCD_RD A0 // LCD Read goes to Analog 0 #define LCD_RESET A4 // Can alternately just connect to Arduino's reset pin #include "Fonts/FreeSans9pt7b.h" #include "Fonts/FreeSans12pt7b.h" #include "Fonts/FreeSerif12pt7b.h" // *** Define Name of Color #define BLACK 0x0000 #define BLUE 0x001F #define RED 0xF800 #define GREEN 0x07E0 #define CYAN 0x07FF #define MAGENTA 0xF81F #define YELLOW 0xFFE0 #define WHITE 0xFFFF #define ORANGE 0xFD20 #define GREENYELLOW 0xAFE5 #define NAVY 0x000F #define DARKGREEN 0x03E0 #define DARKCYAN 0x03EF #define MAROON 0x7800 #define PURPLE 0x780F #define OLIVE 0x7BE0 #define LIGHTGREY 0xC618 #define DARKGREY 0x7BEF #define GREY 0x2108 // Meter colour schemes #define RED2RED 0 #define GREEN2GREEN 1 #define BLUE2BLUE 2 #define BLUE2RED 3 #define GREEN2RED 4 #define RED2GREEN 5 int col[8]; void showmsgXY(int x, int y, int sz, const GFXfont *f, const char *msg) { int16_t x1, y1; uint16_t wid, ht; tft.setFont(f); tft.setCursor(x, y); tft.setTextColor(0xFFE0); tft.setTextSize(sz); tft.print(msg); } uint32_t runTime = -99999; // time for next update int reading = 0; // Value to be displayed int d = 0; // Variable used for the sinewave test waveform boolean alert = 0; int8_t ramp = 1; int tesmod =0; void setup() { // our debugging output Serial.begin(9600); randomSeed(analogRead(0)); tft.reset(); Serial.begin(9600); uint16_t ID = tft.readID(); Serial.println("Example: Font_simple"); Serial.print("found ID = 0x"); Serial.println(ID, HEX); if (ID == 0xD3D3) ID = 0x9481; //force ID if write-only display tft.begin(ID); tft.setRotation(1); tft.fillScreen(BLACK); /////////For showing the loading animation///////// showmsgXY(80, 200, 2, &FreeSans9pt7b, "Initializing..."); col[0] = tft.color565(155, 0, 50); col[1] = tft.color565(170, 30, 80); col[2] = tft.color565(195, 60, 110); col[3] = tft.color565(215, 90, 140); col[4] = tft.color565(230, 120, 170); col[5] = tft.color565(250, 150, 200); col[6] = tft.color565(255, 180, 220); col[7] = tft.color565(255, 210, 240); for (int i = 1; i > 0; i--) { tft.fillCircle(165 + 40 * (cos(-i * PI / 4)), 100 + 40 * (sin(-i * PI / 4)), 10, col[0]); delay(30); tft.fillCircle(165 + 40 * (cos(-(i + 1)*PI / 4)), 100 + 40 * (sin(-(i + 1)*PI / 4)), 10, col[1]); delay(30); tft.fillCircle(165 + 40 * (cos(-(i + 2)*PI / 4)), 100 + 40 * (sin(-(i + 2)*PI / 4)), 10, col[2]); delay(30); tft.fillCircle(165 + 40 * (cos(-(i + 3)*PI / 4)), 100 + 40 * (sin(-(i + 3)*PI / 4)), 10, col[3]); delay(30); tft.fillCircle(165 + 40 * (cos(-(i + 4)*PI / 4)), 100 + 40 * (sin(-(i + 4)*PI / 4)), 10, col[4]); delay(30); tft.fillCircle(165 + 40 * (cos(-(i + 5)*PI / 4)), 100 + 40 * (sin(-(i + 5)*PI / 4)), 10, col[5]); delay(30); tft.fillCircle(165 + 40 * (cos(-(i + 6)*PI / 4)), 100 + 40 * (sin(-(i + 6)*PI / 4)), 10, col[6]); delay(30); tft.fillCircle(165 + 40 * (cos(-(i + 7)*PI / 4)), 100 + 40 * (sin(-(i + 7)*PI / 4)), 10, col[7]); delay(30); } // FOR DISPLAYING TEXT ON THE SCREEN AT VARIOUS POINT// tft.fillScreen(BLACK); //For FAN SPEED in % tft.setCursor (1,212); tft.setTextSize (1); tft.setTextColor (WHITE,BLACK); tft.print ("FAN SPEED"); tft.setCursor (55,55);// For PM 2.5 tft.setTextSize (1); tft.setTextColor (WHITE,BLACK); tft.print ("PM 2.5"); tft.setCursor (215,15); // For PM 1.0 tft.setTextSize (1); tft.setTextColor (WHITE,BLACK); tft.print ("PM 1.0"); tft.setCursor (210,75);//For PM 10.0 tft.setTextSize (1); tft.setTextColor (WHITE,BLACK); tft.print ("PM 10.0"); tft.setCursor (182,135);//For TEMPERATURE tft.setTextSize (1); tft.setTextColor (WHITE,BLACK); tft.print ("TEMPERATURE"); tft.setCursor (202,195);//For HUMIDITY in % tft.setTextSize (1); tft.setTextColor (WHITE,BLACK); tft.print ("HUMIDITY"); //Design Interface (lines) tft.fillRect(0,180,175,3,BLUE); tft.fillRect(0,238,320,3,BLUE); tft.fillRect(175,0,3,240,BLUE); tft.fillRect(317,0,3,240,BLUE); tft.fillRect(175,0,320,3,BLUE); tft.fillRect(175,60,320,3,BLUE); tft.fillRect(175,120,320,3,BLUE); tft.fillRect(175,180,320,3,BLUE); } void loop() { int ringMeter(int value, int vmin, int vmax, int x, int y, int r, char *units, byte scheme) if (millis() - runTime >= 500) { // Execute every 500ms runTime = millis(); if(tesmod==0){reading =5;} if(tesmod==1){reading =2;} int xpos = 0, ypos = 5, gap = 4, radius = 52; // Draw a large meter xpos = 320/2 - 160, ypos = 0, gap = 100, radius = 85; ringMeter(reading,10,100, xpos,ypos,radius,"UM/L",GREEN2RED); // Draw analogue meter ( //Humidity % tft.setCursor (157,208); tft.setTextSize (3); tft.setTextColor (BLUE,BLACK); tft.print (h,0); tft.print ('%'); tesmod=1; } if(f>0){ //Fahrenheit tft.setCursor (237,38); tft.setTextSize (4); tft.setTextColor (BLUE,BLACK); tft.print (f,0); tesmod=1; } if(hif>0){ //Heat index Fahrenheit tft.setCursor (237,138); tft.setTextSize (4); tft.setTextColor (BLUE,BLACK); tft.print (hif,0); tesmod=1; } if(hic>0){ //Heat index Celsius tft.setCursor (238,217); tft.setTextSize (2); tft.setTextColor (BLUE,BLACK); tft.print (hic,0); tesmod=1; } } } // ######################################################################### // Draw the meter on the screen, returns x coord of righthand side // ######################################################################### int ringMeter(int value, int vmin, int vmax, int x, int y, int r, char *units, byte scheme) { // Minimum value of r is about 52 before value text intrudes on ring // drawing the text first is an option x += r; y += r; // Calculate coords of centre of ring int w = r / 4; // Width of outer ring is 1/4 of radius int angle = 150; // Half the sweep angle of meter (300 degrees) int v = map(value, vmin, vmax, -angle, angle); // Map the value to an angle v byte seg = 1; // Segments are 3 degrees wide = 100 segments for 300 degrees byte inc = 1; // Draw segments every 3 degrees, increase to 6 for segmented ring // Variable to save "value" text colour from scheme and set default int colour = BLUE; // Draw colour blocks every inc degrees for (int i = -angle+inc/2; i < angle-inc/2; i += inc) { // Calculate pair of coordinates for segment start float sx = cos((i - 90) * 0.0174532925); float sy = sin((i - 90) * 0.0174532925); uint16_t x0 = sx * (r - w) + x; uint16_t y0 = sy * (r - w) + y; uint16_t x1 = sx * r + x; uint16_t y1 = sy * r + y; // Calculate pair of coordinates for segment end float sx2 = cos((i + seg - 90) * 0.0174532925); float sy2 = sin((i + seg - 90) * 0.0174532925); int x2 = sx2 * (r - w) + x; int y2 = sy2 * (r - w) + y; int x3 = sx2 * r + x; int y3 = sy2 * r + y; if (i < v) { // Fill in coloured segments with 2 triangles switch (scheme) { case 0: colour = GREY; break; // Fixed colour case 1: colour = GREEN; break; // Fixed colour case 2: colour = BLUE; break; // Fixed colour case 3: colour = rainbow(map(i, -angle, angle, 0, 127)); break; // Full spectrum blue to red case 4: colour = rainbow(map(i, -angle, angle, 70, 127)); break; // Green to red (high temperature etc) case 5: colour = rainbow(map(i, -angle, angle, 127, 63)); break; // Red to green (low battery etc) default: colour = BLUE; break; // Fixed colour } tft.fillTriangle(x0, y0, x1, y1, x2, y2, colour); tft.fillTriangle(x1, y1, x2, y2, x3, y3, colour); //text_colour = colour; // Save the last colour drawn } else // Fill in blank segments { tft.fillTriangle(x0, y0, x1, y1, x2, y2, GREY); tft.fillTriangle(x1, y1, x2, y2, x3, y3, GREY); } } // Convert value to a string char buf[10]; byte len = 2; if (value > 999) len = 4; dtostrf(value, len, 0, buf); buf[len] = ' '; buf[len] = 0; // Add blanking space and terminator, helps to centre text too! // Set the text colour to default tft.setTextSize(1); if(value>9){ tft.setTextColor(colour,BLACK); tft.setCursor(x-30,y+25);tft.setTextSize(3); tft.print(buf);} if(value<10){ tft.setTextColor(colour,BLACK); tft.setCursor(x-30,y+25);tft.setTextSize(3); tft.print(buf);} tft.setTextColor(WHITE,BLACK); tft.setCursor(x-20,y+70);tft.setTextSize(1); tft.print(units); // Units display // Calculate and return right hand side x coordinate return x + r; } // ######################################################################### // Return a 16 bit rainbow colour // ######################################################################### unsigned int rainbow(byte value) { // Value is expected to be in range 0-127 // The value is converted to a spectrum colour from 0 = blue through to 127 = red byte red = 0; // Red is the top 5 bits of a 16 bit colour value byte green = 0;// Green is the middle 6 bits byte blue = 0; // Blue is the bottom 5 bits byte quadrant = value / 32; if (quadrant == 0) { blue = 31; green = 2 * (value % 32); red = 0; } if (quadrant == 1) { blue = 31 - (value % 32); green = 63; red = 0; } if (quadrant == 2) { blue = 0; green = 63; red = value % 32; } if (quadrant == 3) { blue = 0; green = 63 - 2 * (value % 32); red = 31; } return (red << 11) + (green << 5) + blue; } // ######################################################################### // Return a value in range -1 to +1 for a given phase angle in degrees // ######################################################################### float sineWave(int phase) { return sin(phase * 0.0174532925); }
And if you want to download the CODE ,you can click on this link for that
final_tft_program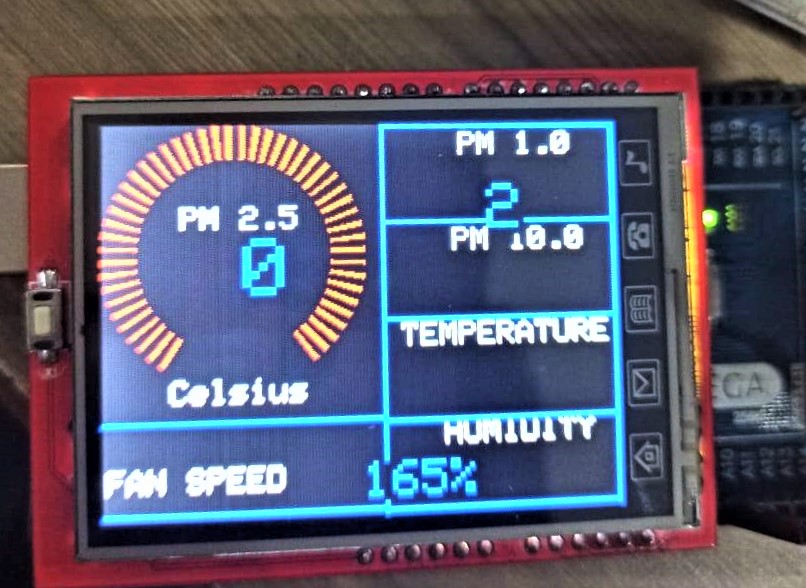
From this code you can see that how this code works , this code consist of the this meter as well as the loading part which i have shown you above this was my original code